Two JVM options are often used to tune JVM heap size: -Xmx for maximum heap size, and -Xms for initial heap size. Here are some common mistakes I have seen when using them:
• Missing m, M, g or G at the end (they are case insensitive). For example,
java -Xmx128 TestProgram
java.lang.OutOfMemoryError: Java heap space
The correct command should be: java -Xmx128m TestProgram. To be precise, -Xmx128 is a valid setting for very small apps, like HelloWorld. But in real life, I guess you really mean -Xmx128m
• Extra space in JVM options, or incorrectly use =. For example,
java -Xmx 128m TestProgram
Invalid maximum heap size: -Xmx
Could not create the Java virtual machine.
java -Xmx=512m HelloWorld
Invalid maximum heap size: -Xmx=512m
Could not create the Java virtual machine.
The correct command should be java -Xmx128m TestProgram, with no whitespace nor =. -X options are different than
-Dkey=value system properties, where = is used.
• Only setting -Xms JVM option and its value is greater than the default maximum heap size, which is 64m. The default minimum heap size seems to be 0. For example,
java -Xms128m TestProgram
Error occurred during initialization of VM
Incompatible initial and maximum heap sizes specified
The correct command should be java -Xms128m -Xmx128m TestProgram. It's a good idea to set the minimum and maximum heap size to the same value. In any case, don't let the minimum heap size exceed the maximum heap size.
• Heap size is larger than your computer's physical memory.For example,
java -Xmx2g TestProgram
Error occurred during initialization of VM
Could not reserve enough space for object heap
Could not create the Java virtual machine.
The fix is to make it lower than the physical memory: java -Xmx1g TestProgram
• Incorrectly use mb as the unit, where m or M should be used instead.
java -Xms256mb -Xmx256mb TestProgram
Invalid initial heap size: -Xms256mb
Could not create the Java virtual machine.
• The heap size is larger than JVM thinks you would ever need. For example,
java -Xmx256g TestProgram
Invalid maximum heap size: -Xmx256g
The specified size exceeds the maximum representable size.
Could not create the Java virtual machine.
The fix is to lower it to a reasonable value: java -Xmx256m TestProgram
• The value is not expressed in whole number. For example,
java -Xmx0.9g TestProgram
Invalid maximum heap size: -Xmx0.9g
Could not create the Java virtual machine.
The correct command should be java -Xmx928m TestProgram
How to set java heap size in Eclipse?
You have 2 options:
1. Edit eclipse-home/eclipse.ini to be something like the following and restart Eclipse.
-vmargs
-Xms64m
-Xmx256m
2. Or, you can just run eclipse command with additional options at the very end. Anything after -vmargs will be treated as JVM options and passed directly to the JVM. JVM options specified in the command line this way will always override those in eclipse.ini. For example,
eclipse -vmargs -Xms64m -Xmx256m
Subscribe to:
Post Comments (Atom)
Heroku Custom Trust Store for SSL Handshake
Working with Heroku for deploying apps (java, nodejs, etc..) is made very easy but while integrating one of the service ho...
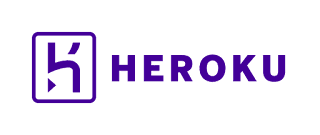
-
Multi-Tenancy with the SaaS based model does not bring any change on the application layer as multiple services deployment of application w...
-
Raspberry Pi is an awesome and interesting . I was totally astonished after knowing about it from one of my friend and colleague "Ale...
-
I have a class which is not serializable. If i will try to serialize the object of it than i am going to get exception "java.io.NotSer...
No comments:
Post a Comment